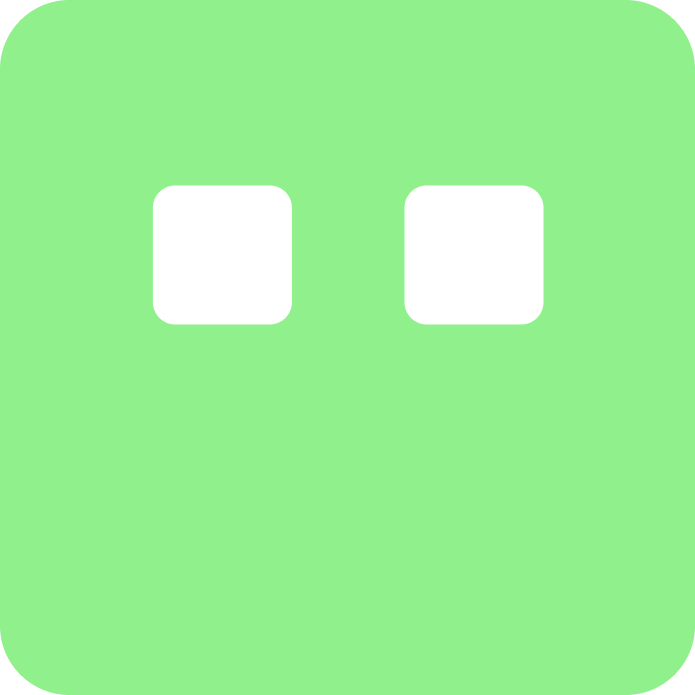
Limeblock Docs
Getting Started with Limeblock
Welcome to Limeblock! This guide will walk you through the process of setting up your Limeblock chat widget from account creation to final implementation. Follow these steps to add an AI-powered chat experience to your application.
Step 1: Create Your Limeblock Account
First, you'll need to create an account on the Limeblock platform:
- Visit limeblock.io
- Click on "Sign Up" and follow the registration process
- Add your email address + team members
- Choose a plan or upgrade when ready
Tip: For team environments, consider setting up a shared organizational account that multiple team members can access. You can add multiple emails in your settings or when you create an account.
Step 2: Create a New Limeblock Widget
Once your account is set up, you'll need to create a widget. Add a button to your application's admin interface to quickly access the creation page:
// Give the widget the color, size, and location you want in Limeblock - no code needed
When creating your widget, you'll need to:
- Give your widget a name (e.g., "Help Assistant")
- Select the primary function (customer support, documentation, etc.)
- Choose an initial template or start from scratch
- Configure the knowledge base sources
Step 3: Style Your Widget in the Frontend Page
Limeblock offers comprehensive styling options directly in the dashboard. You can customize:
- Chat bubble colors and positioning
- Font styles and sizes
- Widget header and footer appearance
- Custom CSS overrides for advanced styling
- Mobile and desktop responsive behaviors
All styling is managed through the Limeblock dashboard - no custom code needed! This approach ensures consistent updates and easier maintenance.
Step 4: Set Up Page Navigations
Configure how your widget interacts with your application's pages:
// Example of page navigation configuration in the Limeblock app { "pages": [ { "title": "Home", "path": "/", "description": "Welcome page with introduction" }, { "title": "Documentation", "path": "/docs", "description": "Help documentation and guides" }, { "title": "Settings", "path": "/settings", "description": "Configure your Limeblock settings" } ] }
In the Limeblock dashboard:
- Navigate to the "Navigation" tab
- Add each page in your application
- Configure when and how the widget suggests navigations
- Set up transition animations between pages
- Test the navigation flows before publishing
Step 5: Set Up Backend API Endpoints
For full functionality, you'll need to set up backend endpoints:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
// Example JSON schema provided Limeblock backend integration // For the API Endpoint - Create Board const url = {https://example_endpoint.com/api/create_board/} // Schema with context params and fully filled in // This an example template for the AI to use const schema = { "settings": { "borders": "#FFFFFF", "boardBackground": "#FFFFFF", "pageBackground": "#F1F1F1", "titleColor": "#000000", "subtitleColor": "#000000", "dateRange": "#333333", "tableHeaders": "#0b0b0b", "ranks": "#333333", "rankingField": "#333333", "nameField": "#333333", "title": "My Limeblock Dashboard", "subtitle": "Real-time data visualization", "rankingTitle": "Score", "nameTitle": "User", "titleFont": "'Roboto', sans-serif", "subtitleFont": "'Roboto', sans-serif", "boardRankTitleFont": "'Roboto', sans-serif", "boardRankFont": "'Roboto', sans-serif", "boardNameTitleFont": "'Roboto', sans-serif", "boardNameFont": "'Roboto', sans-serif" }, "board_id": "{board_id}", "user_id": "{user_id}" };
Important Note: You'll need to give Limeblock access to your backend by following the instructions in the Backend Integration page of the dashboard. This includes setting up proper authentication and defining the allowed scope of operations.
Step 6: Get Your API Key
To connect your application with Limeblock, you'll need your API key:
- Go to the Settings page in your Limeblock dashboard
- Navigate to the "API Keys" section
- Generate a new API key (or use an existing one)
- Copy the key and store it securely as an environment variable
// Using your API key from settings const LIMEBLOCK_API_KEY = process.env.NEXT_PUBLIC_LIMEBLOCK_API_KEY || "lime_YOUR_API_KEY";
Step 7: Export and Implement Your Widget
Follow the Export documentation to implement the widget in your application:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
import React from 'react'; import { ChatWidget } from '@limeblock/react'; const MyApp = () => { // Your API key from Limeblock settings const API_KEY = process.env.NEXT_PUBLIC_LIMEBLOCK_API_KEY; // Context parameters configured in Limeblock dashboard const contextParams = { board_id: "YOUR_BOARD_ID_FROM_DASHBOARD", user_id: "current_user_id", // Dynamic user identification // Additional context parameters as needed }; return ( <div className="my-app-container"> {/* Your application content */} <main> <h1>Welcome to My Application</h1> <p>This is where your content lives</p> </main> {/* Limeblock Chat Widget */} <ChatWidget apiKey={API_KEY} contextParams={contextParams} // All styling is configured in the Limeblock dashboard /> </div> ); }; export default MyApp;
Important Note: If you are using NextJS please add "use client"; to the top of the page
For more detailed implementation instructions, please refer to our Export Documentation.
Troubleshooting
Widget not appearing
Check your API key and ensure the widget is published in the dashboard.
Backend connection issues
Verify your webhook endpoints are configured correctly and accessible to Limeblock's servers.
Navigation not working
Ensure your page paths in the Limeblock dashboard exactly match your application's routing structure.
Getting Help
If you need assistance with your Limeblock implementation:
- Contact our founder directly at byjuaditya@gmail.com for personalized support
- Check our comprehensive documentation