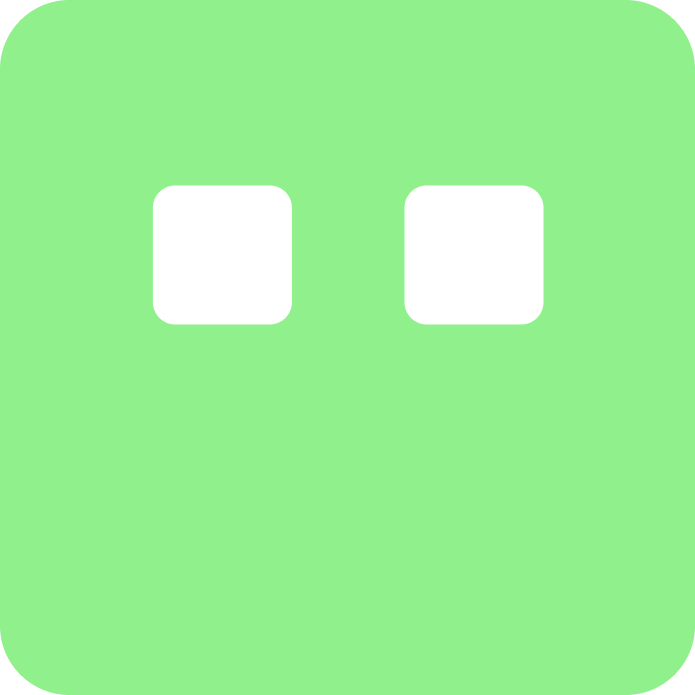
Limeblock Docs
Endpoint Tree Docs
This guide will walk you through the process of configuring your backend to work seamlessly with Limeblock. Proper backend integration enables your Limeblock AI endpoints to access data, perform actions, and provide a more personalized experience to your users.
Backend URL Configuration
The first step in backend integration is to specify your API's base URL:
- Navigate to the Backend tab in your Limeblock dashboard
- Enter your API's base URL in the "Edit URL" section (e.g.,
https://api.yourapp.com
) - Save the configuration
API Endpoint Configuration
Limeblock can interact with your backend through the API endpoints you defined in app. Follow these guidelines to configure them effectively:
- Organize with Folders: Group related endpoints in logical folders (e.g., "User Management", "Content", "Transactions")
- Define Clear Paths: Use RESTful paths that clearly indicate the endpoint's purpose
- Provide Detailed Descriptions: Write comprehensive descriptions that help the AI understand when and how to use each endpoint
Example API Endpoint Structure
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
{ "folders": [ { "name": "User Management", "endpoints": [ { "path": "/api/users/profile", "method": "GET", "description": "Retrieves the current user's profile information including name, email, preferences, and account status. Returns a 404 if the user is not found or a 401 if unauthorized.", "schema": { "user_id": "{user_id}" } }, { "path": "/api/users/update", "method": "PUT", "description": "Updates the user's profile information. Can modify name, email, and preferences. Returns the updated user object or errors for invalid inputs.", "schema": { "user_id": "{user_id}", "name": "string", "email": "string", "preferences": { "notifications": "boolean", "theme": "string" } } } ] }, { "name": "Products", "endpoints": [ { "path": "/api/products/list", "method": "GET", "description": "Retrieves a paginated list of products with optional filtering by category, price range, and availability. Returns product information including ID, name, price, and description.", "schema": { "category": "string", "min_price": "number", "max_price": "number", "in_stock": "boolean", "page": "number", "limit": "number" } }, { "path": "/api/products/{product_id}", "method": "GET", "description": "Retrieves detailed information about a specific product including features, specifications, pricing, and availability. Returns a 404 if the product is not found.", "schema": { "product_id": "{product_id}" } } ] } ] }
Best Practices for Endpoint Descriptions
Write detailed descriptions that include:
- What the endpoint does
- What parameters it accepts
- What response it returns
- Potential error cases
- Use cases for when this endpoint should be called
Example of a Good Description:
Creates a new order in the system with the provided items and shipping information. Validates inventory availability and calculates final price including taxes and shipping. Returns the created order ID and confirmation details, or specific error messages if validation fails (e.g., insufficient inventory, invalid shipping address).
Best Practices for Instructions
Effective instructions should include:
- Clear and concise language
- Step-by-step breakdown of actions
- Expected outcomes or goals
- Important warnings or tips to avoid potential errors
- Examples to clarify complex situations
- Desired structure of schema when sending (example- send the whole schema context or just the part meant to be updated)
Example of Good Instructions:
You can leave the name field blank. Make sure to send the entire schema structure including the context, not just the new part. Create unique ids.
Schema Instructions
Proper schema definition is crucial for successful AI integration. Follow these guidelines to ensure your schemas are correctly formatted:
Context Parameters Formatting
- Wrap in curly braces: Context parameters must be wrapped in curly braces (e.g.,
"{userId}"
) - Exact casing: Use the exact casing your backend expects (camelCase, snake_case, etc.)
- Consistency: Maintain the same casing throughout your schema and implementation
- No spaces: Avoid spaces in parameter names (use
timePeriod
instead oftime period
)
Data Format Guidelines
Use actual values in your schema examples to demonstrate expected formats:
Data Type | Format Example | Notes |
---|---|---|
String | "ORD-78945" | Use quotes for all string values |
Number | 149.99 | No quotes for numbers |
Boolean | true or false | No quotes, lowercase |
Array | [{...}] | Contains actual objects with values |
Object | {...} | Nested structures with actual values |
Schema Example with Actual Values
Here's an example schema demonstrating correct casing, context parameters, and actual values:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
// Example of well-defined schema with actual values { "userId": "{userId}", // Context parameter in correct casing "orderDetails": { "orderId": "ORD-78945", // Actual string value "totalAmount": 149.99, // Actual number value "isCompleted": true, // Actual boolean value "items": [ // Array with actual values { "productId": "PROD-123", "quantity": 2, "unitPrice": 59.99 }, { "productId": "PROD-456", "quantity": 1, "unitPrice": 29.99 } ], "shippingAddress": { // Nested object with actual values "street": "123 Elm Street", "city": "San Francisco", "zipCode": "94105" } }, "preferences": { "notificationOptIn": true, "preferredTheme": "dark" } }
Important: This example shows the exact structure and value types that would be sent to your endpoint. The AI will generate requests matching this format exactly. Context parameters like "{userId}"
will be replaced with actual values at runtime.
Context Implementation Consistency
When providing context in your implementation, the casing must match exactly what you defined in your schema:
1 2 3 4 5 6 7 8 9 10 11 12 13
// When making API requests from your app const context = { userId: "user_12345", // Must match casing exactly timePeriod: "30 days", // Must match casing includeArchived: true // Must match casing }; // This will be sent to the endpoint as: { "userId": "user_12345", "timePeriod": "30 days", "includeArchived": true }
Warning: Casing mismatches will cause context parameters to not be replaced properly.userId
and userid
are considered different parameters. This is the most common integration error.
Configuring Backend Access
For Limeblock to communicate with your backend, you need to set up proper access controls:
CORS Configuration
Configure your server to accept requests from Limeblock's domains by adding appropriate CORS (Cross-Origin Resource Sharing) headers.
Django Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
# settings.py INSTALLED_APPS = [ # ... other apps 'corsheaders', ] MIDDLEWARE = [ 'corsheaders.middleware.CorsMiddleware', # ... other middleware ] # Allow requests from Limeblock domains CORS_ALLOWED_ORIGINS = [ "https://limeblock.io", "https://limeblockbackend.onrender.com", ] # If you need to allow credentials (cookies, authorization headers) CORS_ALLOW_CREDENTIALS = True # Optional: Specify which HTTP methods are allowed CORS_ALLOW_METHODS = [ "GET", "POST", "PUT", "PATCH", "DELETE", "OPTIONS" ]
Express.js Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
const express = require('express'); const cors = require('cors'); const app = express(); // Configure CORS app.use(cors({ origin: [ "https://limeblock.io", "https://limeblockbackend.onrender.com", ], credentials: true, methods: ['GET', 'POST', 'PUT', 'DELETE', 'OPTIONS'], allowedHeaders: ['Content-Type', 'Authorization'] })); // Your routes and other middleware
Authentication
To secure your API endpoints, consider implementing one of these authentication methods:
- API Key Authentication: Include your Limeblock API key in requests
- OAuth 2.0: For more advanced security needs
- JWT Tokens: For stateless authentication
Testing Your Backend Integration
Before deploying to production:
- Use the "Test Endpoint" feature (With the edit and delete buttons) in the Limeblock endpoint preview box to verify connectivity
- Test with various inputs to ensure robust error handling
- Verify context parameter substitution works correctly
- Check that the AI can properly interpret the responses
Troubleshooting
Issue | Potential Solution |
---|---|
403 Forbidden errors | Check CORS configuration and authentication |
Endpoint not being called | Verify URL path and description accuracy |
Context parameters not being substituted | Ensure parameter names match in schema and implementation |
AI misinterpreting responses | Improve endpoint descriptions and provide example responses |
Getting Help
If you need assistance with your Limeblock implementation:
- Contact our founder directly at byjuaditya@gmail.com for personalized support
- Check our comprehensive documentation